Vue.js Lifecycle Hooks
Vue.js Lifecycle Hooks
Vue.js is a popular JavaScript framework used for building dynamic user interfaces. It offers a powerful set of tools for developers to create highly interactive and responsive applications. One of the key features of Vue.js is its component-based architecture, which allows developers to build complex applications using reusable and modular code blocks.
In this blog post, we will discuss the Vue.js lifecycle hooks and how they can be used to manage the behavior of components at different stages of their life cycle.
Vue.js has a set of lifecycle hooks that allow developers to run code at different stages of a component's life cycle. These hooks provide developers with a way to perform operations when a component is created, mounted, updated, or destroyed. There are eight different lifecycle hooks available in Vue.js, and they are as follows:
-
beforeCreate:
This hook is called before the component is created. It is useful for setting up component data, initializing variables, and setting up event listeners.
-
created:
This hook is called after the component has been created. It is useful for performing any operations that require access to the component's data and methods.
-
beforeMount:
This hook is called before the component is mounted. It is useful for performing any last-minute preparations before the component is rendered.
-
mounted:
This hook is called after the component has been mounted. It is useful for performing any operations that require access to the component's DOM.
-
beforeUpdate:
This hook is called before the component is updated. It is useful for performing any operations that need to be done before the component's data changes.
-
updated:
This hook is called after the component has been updated. It is useful for performing any operations that need to be done after the component's data changes.
-
beforeDestroy:
This hook is called before the component is destroyed. It is useful for performing any clean-up operations before the component is removed from the DOM.
-
destroyed:
This hook is called after the component has been destroyed. It is useful for performing any final clean-up operations.
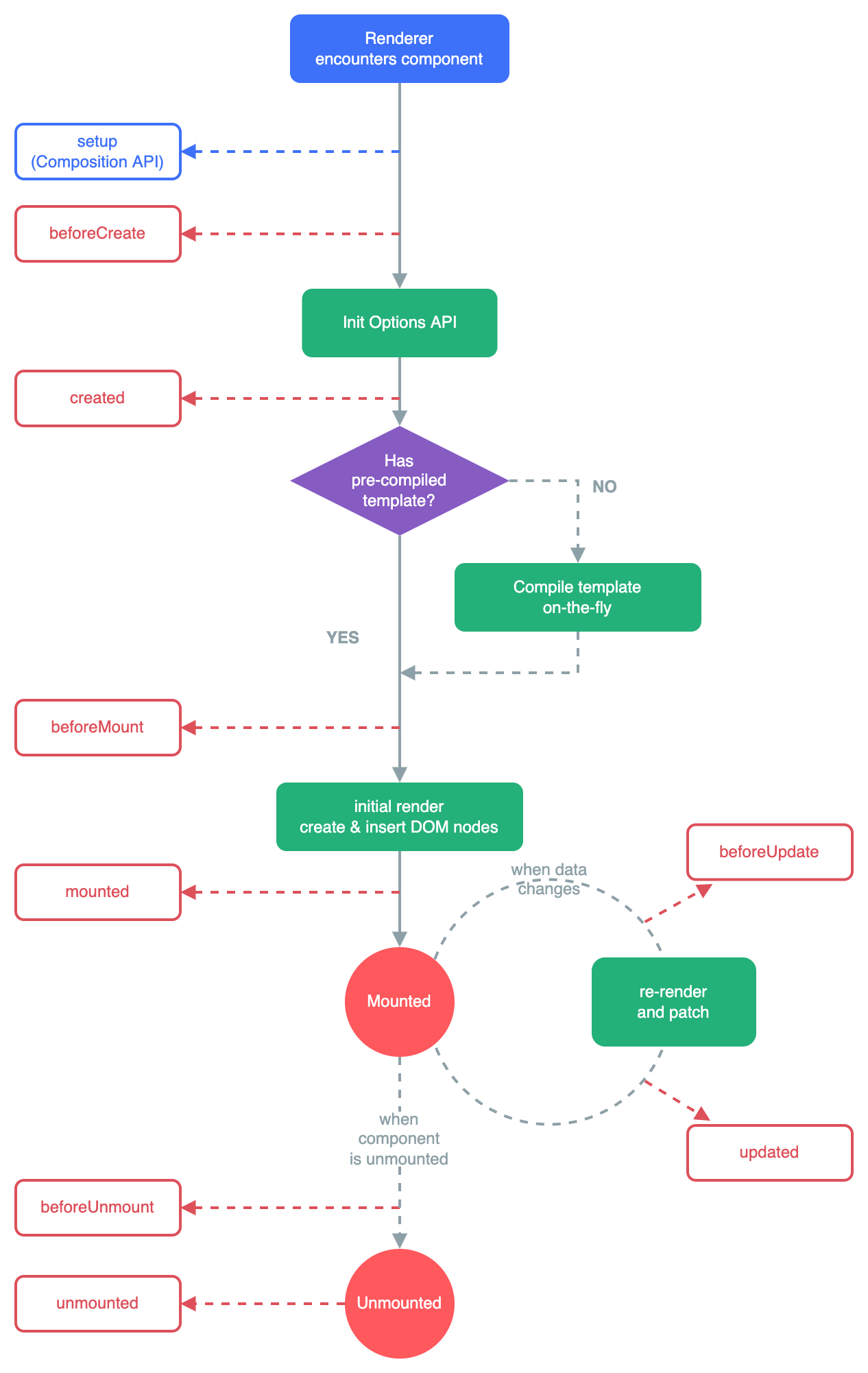
Let's take a look at an example of how these hooks can be used to manage the behavior of a component:
// Vue component example
<template>
<div>
<h1>{{ message }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
message: "Hello, world!",
};
},
beforeCreate() {
console.log("Before Create");
},
created() {
console.log("Created");
},
beforeMount() {
console.log("Before Mount");
},
mounted() {
console.log("Mounted");
},
beforeUpdate() {
console.log("Before Update");
},
updated() {
console.log("Updated");
},
beforeDestroy() {
console.log("Before Destroy");
},
destroyed() {
console.log("Destroyed");
},
};
</script>
In the above example, we have defined a simple component that displays a message on the screen. We have also included all the available lifecycle hooks in the component.
When the component is created, Vue.js will call the `beforeCreate` hook followed by the `created` hook. Then, it will call the `beforeMount` hook and mount the component to the DOM by calling the `mounted` hook. If the data of the component changes, Vue.js will call the `beforeUpdate` hook followed by the `updated` hook. Finally, when the component is destroyed, Vue.js will call the `beforeDestroy` hook followed by the `destroyed` hook.